In this post we will see the difference between StatelessWidget and StefulWidget and when to use them.
Widgets in Flutter
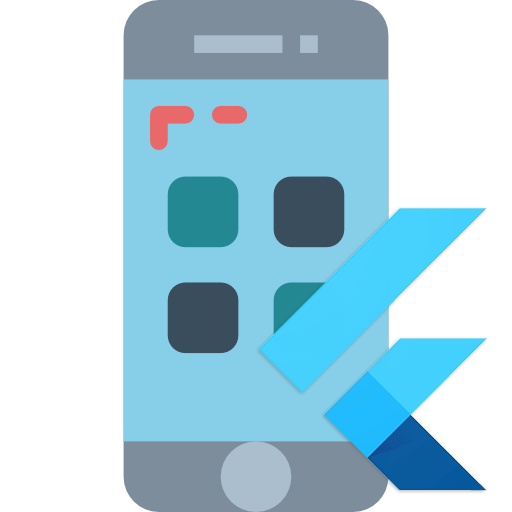
In Flutter Widgets are used to create a modern user interface in a few minutes. Widgets are the key component of any app developed in Flutter.
Widgets describe how their appearance must be with respect to the their configuration and state.
Every time the state of a widget changes it rebuild its view with respect to the state it is in.
Unlike other frameworks, an App developed in Flutter the UI is not completely redrawn when a state changes, but only the UI of the widget to which the state belongs.
The complete list of all Widgets is at the following link https://flutter.dev/docs/development/ui/widgets
The two types of widget
In Flutter a widget can be Stateless or Stateful. Let’s take a look at the difference:
Stateless Widget
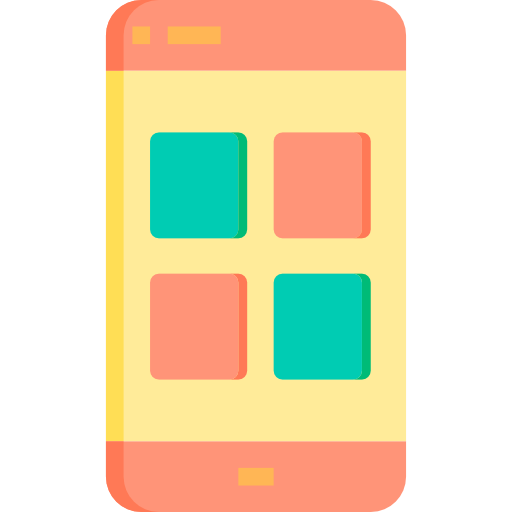
“Stateless” mean without a state, so a Stateless widget can’t change its elements.
Examples of StatelessWidget are Text, Icon e IconButton.
import 'package:flutter/material.dart';
//the first function called at runtime
void main() {
runApp(MyApp());
}
//implement the StatelessWidget
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'My Super Awesome App',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: Scaffold(
appBar: AppBar(
title: Text("La mia bellissima App"),
),
body: Center(child:Text('My Super Awesome App!'))
),
);
}
}
As we can see from the code, we have developed a simple App with a static text centered in the screen that never changes.
Stateful Widget
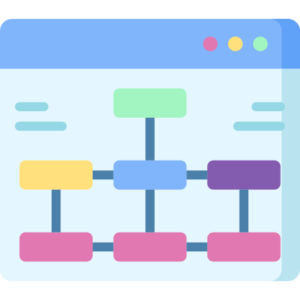
Unlike the previous one, a Stateful Widget is dynamic: it changes its appearance every time that user trigger an event or when it receives data.
Examples of StatefulWidget are Checkbox, Radio, Slider, Form e TextField.
In the following code we implement a Stateful widget that increments a counter by pressing a FloatingActionButton:
import 'package:flutter/material.dart';
//the first function called at runtime
void main() {
runApp(MyHomePage());
}
//implement the StateFulWidget
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState() => _MyHomePageState();
}
//implement the Widget state
class _MyHomePageState extends State {
int _counter = 0;
//the function to incement the counter
void _incrementCounter() {
//with setState() we trigger the state change of the widget
setState(() {
_counter++;
});
}
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'My Super Awesome App',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: Scaffold(
appBar: AppBar(
title: Text("My Super Awesome App"),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text('You have pushed the button:',),
Text('$_counter', style: Theme.of(context).textTheme.headline4,),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: Icon(Icons.add),
),
),);
}
}
As we can see from the code, to trigger the state change and to redraw the widget interface we need to call the setState() function and change the value of the variables that describes the widget.
Practical example: A quiz App
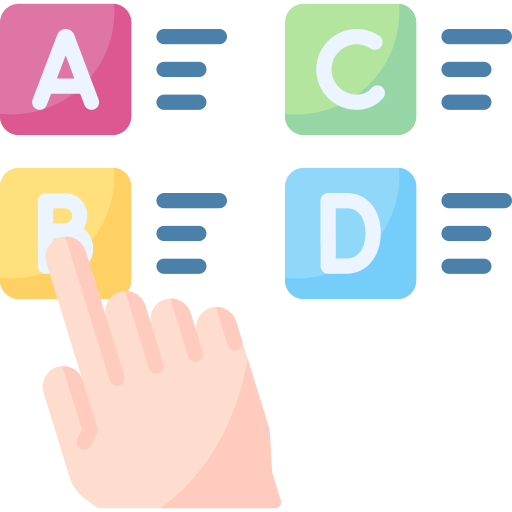
Let’s take a look to a practical example: a quiz App.
In this case we can have x questions. For each question we have to store the answer and keep going with the next one or we have to show the final result.
Which widget we need for this purpose?
In this case we need a StatefulWidget: every time the user answer a question we have to call the setState() function and store the answer, computer the score and increment the counter to skip to the next question.